API Documentation (GQL PAPI)
Manage ReadMe information, Spotlights, Tutorials and/or Assets to your API.
For an overview of general documentation options for your API, see Documenting Your API.
For reading and updating an API's Description, see Reading API Information (GQL PAPI) and REST APIs (GQL PAPI).
For reading and updating an API's Long Description , see Reading API Information (GQL PAPI) and REST APIs (GQL PAPI).
Working with ReadMe / Docs
For an overview of ReadMe / Docs, see Hub Listing - Docs Tab.
Read an API's ReadMe
Use query.api.documentation.readme
. See Reading API Information (GQL PAPI).
Add, update, or delete an API's ReadMe
Use the text
variable to specify the ReadMe text. Set it to "" to delete the ReadMe section on the About tab.
mutation createDocumentation($input: DocumentationCreateInput!) {
createDocumentation(input: $input)
}
{
"input": {
"apiId": "api_64fb3e9b-762c-40df-b561-1adf4ce9890c",
"readme": "My **Readme** information."
}
}
Working with Spotlights
For an overview of Spotlights, see Spotlights.
Read an API's Spotlights
Use query.api.documentation.spotlights
. See Reading API Information (GQL PAPI). Also, query.spotlights
can be used.
Add a Spotlight to an API
mutation CreateSpotlight($spotlight: SpotlightCreateInput!) {
createSpotlight(spotlight: $spotlight) {
id
}
}
{
"spotlight": {
"type": "link",
"description": "Spotlight 2",
"spotlightURL": "https://yahoo.com",
"title": "Spotlight 2",
"published": true,
"apiId": "api_b766b545-cd34-4b8a-8ec9-0e994cf6ed80",
"weight": 1
}
}
Update an API's Spotlight
mutation UpdateSpotlight($spotlight: SpotlightUpdateInput!) {
updateSpotlight(spotlight: $spotlight) {
id
}
}
{
"spotlight": {
"description": "Spotlight Updated",
"title": "Spotlight Updated",
"apiId": "api_b766b545-cd34-4b8a-8ec9-0e994cf6ed80",
"weight": 1,
"id": "10489"
}
}
Delete an API's Spotlight
mutation DeleteSpotlight($id: ID!, $apiId: ID!) {
deleteSpotlight(spotlight: { id: $id, apiId: $apiId })
}
{
"id": "10489",
"apiId": "api_b766b545-cd34-4b8a-8ec9-0e994cf6ed80"
}
Working with Tutorials
For an overview of Tutorials, see Tutorials.
Read an API's Tutorials
To read an API's tutorials, you must specify the API ID and version ID in the variables.
query tutorials($id: ID!, $versionId: ID!) {
tutorials(where: { apiId: $id, apiVersion: $versionId }) {
nodes {
id
slugifiedName
published
title
content
thumbnailURL
publishedDate
readTime
__typename
}
__typename
}
}
{
"id": "api_b766b545-cd34-4b8a-8ec9-0e994cf6ed80",
"versionId": "apiversion_1de8cd3a-94ec-45f0-b193-8d6f9a04dcd4"
}
Add a Tutorial to an API
mutation CreateTutorial(
$id: ID!
$versionId: ID!
$title: String!
$content: String!
$published: Boolean!
$thumbnailURL: String
) {
createTutorial(
tutorial: {
apiId: $id
apiVersion: $versionId
title: $title
content: $content
published: $published
thumbnailURL: $thumbnailURL
}
) {
id
}
}
{
"id": "api_b766b545-cd34-4b8a-8ec9-0e994cf6ed80",
"versionId": "apiversion_1de8cd3a-94ec-45f0-b193-8d6f9a04dcd4",
"title": "Tutorial 2",
"content": "**Tutorial** content.",
"published": true
}
Update an API's Tutorial
mutation UpdateTutorial(
$id: ID!
$versionId: ID!
$title: String
$content: String
$published: Boolean
$thumbnailURL: String
$slug: String!
) {
updateTutorial(
tutorial: {
apiId: $id
apiVersion: $versionId
title: $title
content: $content
published: $published
thumbnailURL: $thumbnailURL
slugifiedName: $slug
}
) {
id
slugifiedName
}
}
{
"id": "api_b766b545-cd34-4b8a-8ec9-0e994cf6ed80",
"versionId": "apiversion_1de8cd3a-94ec-45f0-b193-8d6f9a04dcd4",
"slug": "tutorial-2",
"published": true,
"title": "Tutorial 2",
"content": "**Tutorial** content updated.",
"thumbnailURL": null
}
Delete an API's Tutorial
mutation DeleteTutorial($id: ID!, $versionId: ID!, $slug: String!) {
deleteTutorial(
tutorial: { apiId: $id, apiVersion: $versionId, slugifiedName: $slug }
)
}
{
"id": "api_b766b545-cd34-4b8a-8ec9-0e994cf6ed80",
"versionId": "apiversion_1de8cd3a-94ec-45f0-b193-8d6f9a04dcd4",
"slug": "tutorial-2"
}
Working with Assets
For an overview of Assets, see Hub Listing - Assets Tab.
Read an API's Assets
You can use query.apiVersion
to obtain a list of an API version's Assets. In the Variables, you must specify the API version ID. In the response, the Asset's id
is returned. You can use this Asset ID to modify the Asset using the mutations listed below.
query ApiVersion ($id: ID!) {
apiVersion(apiVersionId: $id) {
id
assets {
id
filename
title
visible
description
fileSizeBytes
}
}
}
{
"id": "apiversion_d071dfc7-5711-48e9-91f6-6354a6ec659c"
}
Add an Asset to an API
To add assets to an API via the GraphQL Platform API, you use the generateAssetUploadUrl
and updateAssetUploaded
mutations, as shown in the Node.js sample code shown below.
You must specify the API version ID, which identifies the API and version for which you want to upload assets. You can obtain the version ID programmatically or using the Studio interface. Choose Hub Listing > Endpoints > (select your version) > OpenAPI for the API and copy the API Version ID (see below).
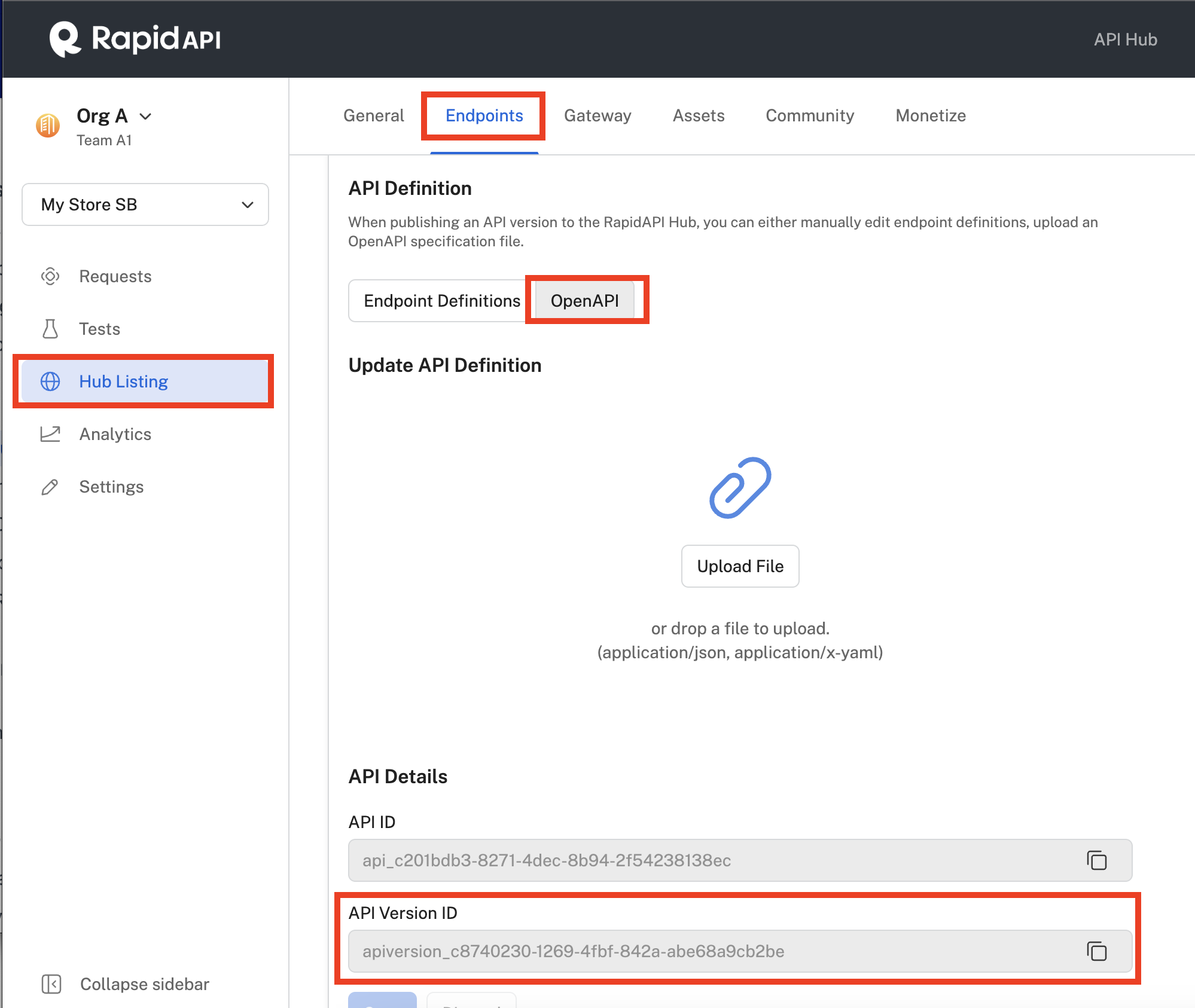
const axios = require("axios");
const PlatformAPIUrl = 'YOUR URL';
const XRapidAPIKey = 'YOUR KEY';
const XRapidAPIHost = 'YOUR HOST';
const ApiVersionId = 'YOUR VERSION ID';
const title = 'YOUR FILE TITLE';
const file = 'YOUR FILE NAME';
const generateAssetResponseOptions = {
method: 'post',
url: PlatformAPIUrl,
headers: {
'content-type': 'application/json',
'X-RapidAPI-Key': XRapidAPIKey,
'X-RapidAPI-Host': XRapidAPIHost
},
data: {
query: `mutation generateAssetUploadUrl($input: GenerateAssetUploadUrlInput!) {
generateAssetUploadUrl(input: $input) {
id
presignedUrl
}
}`,
"variables": {
"input" : {
"title": title,
"filename": file,
"externalId": ApiVersionId
}
}
}
};
const updateAssetUploadedOptions = (id) => {
return {
method: 'post',
url: PlatformAPIUrl,
headers: {
'content-type': 'application/json',
'X-RapidAPI-Key': XRapidAPIKey,
'X-RapidAPI-Host': XRapidAPIHost
},
data: {
query: `mutation updateAssetUploaded($id: ID!) {
updateAssetUploaded(id: $id) {
id
}
}`,
"variables": { "id" : id }
}
};
}
// Send a request to generate an asset object and recieve a url to upload the file to
axios.request(generateAssetResponseOptions).then(async ({data}) => {
// Upload the file to the presignedUrl
await axios.put(data.data.generateAssetUploadUrl.presignedUrl, {"testJson": "testJson"});
// Set the asset to uploaded state once the upload was finished succcessfully
await axios.request(updateAssetUploadedOptions(data.data.generateAssetUploadUrl.id));
}).catch((err) =>{
console.error(err)
});
Update an API's Asset
Use mutation.updateAsset
to update an existing Asset's metadata, such as its title, description, or visibility. In the Variables, you must specify the Asset ID, which can be found using query.apiVersion
(described above).
mutation updateAsset($input: AssetUpdateInput!) {
updateAsset(input: $input) {
id
title
description
visible
__typename
}
}
{
"input": {
"id": "052a4ce6-8767-43f7-8906-e519a787fac4",
"title": null,
"description": "Asset description.",
"visible": true
}
}
Delete an API's Asset
Use mutation.deleteAsset
. TBD
Updated about 1 month ago