JSON Schema Validation
Why validate your JSON response?
In modern software development architecture, APIs are often used as an interface for two systems to talk to each other. They can be used for frontend systems to communicate with backend logic or simply for two backend systems to talk to each other.
Given that an API is a means of communication, a contract exists between the API builder and the consumer on how the API will provide information. For the consumers to consume information seamlessly, the API response must always follow the API contract. Therefore, it is crucial to constantly monitor whether API responses meet the contract and whether a code change deployed to production would break the contract before deploying the change.
How to validate a JSON Response in RapidAPI Testing
RapidAPI Testing allows you to quickly validate your API responses against the schema it must abide by. To do this, you will need the response from an API call (you can make the HTTP request in the test itself) and the JSON Schema (more on JSON Schema) we will validate against.
First, letโs add the HTTP request whose response you will validate. The example below is simply a GET request to https://mystoreapi.com/catalog/products
. We will store the response in a variable called getProductsResponse
.
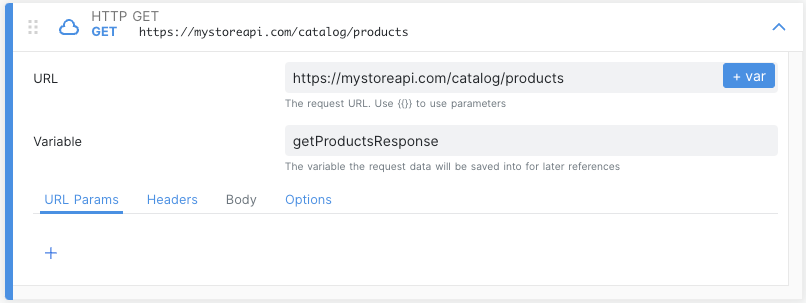
Next, add a JSON Validate step to your test.
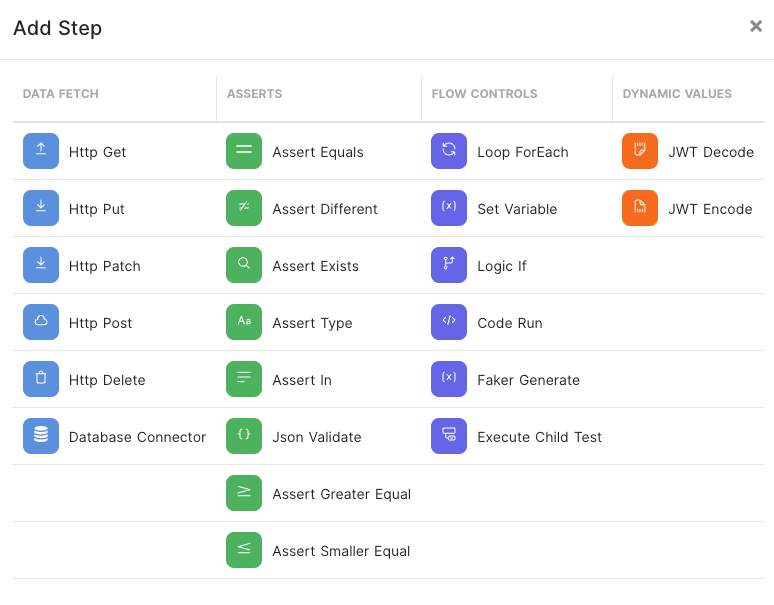
In the JSON Validate step, select the test variable that holds the JSON data you want to validate against (box 1). In this example, you want to validate the JSON in getProductsResponse.data.products
. The JSON schema you will validate against goes in the JSON schema editor (box 2). The schema editor helps you by letting you know whether the schema is valid. (More on JSON Schema).
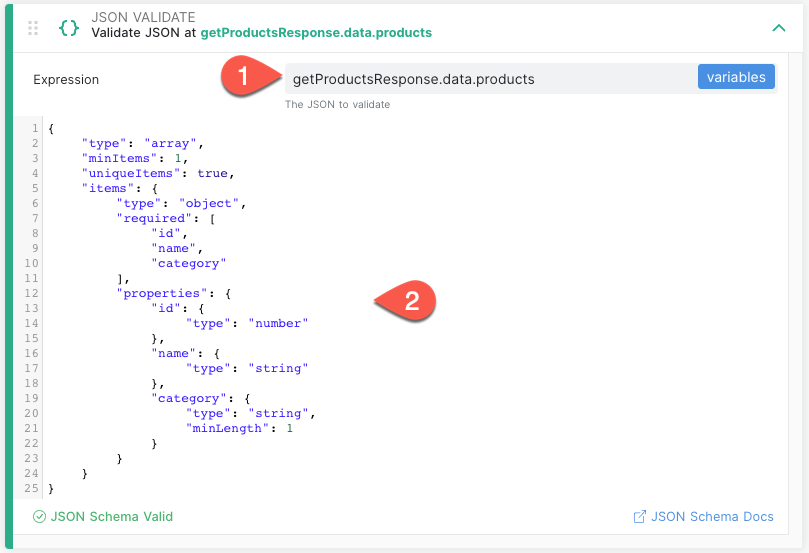
Now execute the test. The execution report will let you know whether the JSON data provided passes the validation. If it does not, the details will explain why. In the example below, the category field must have at least one character, but itโs an empty string.
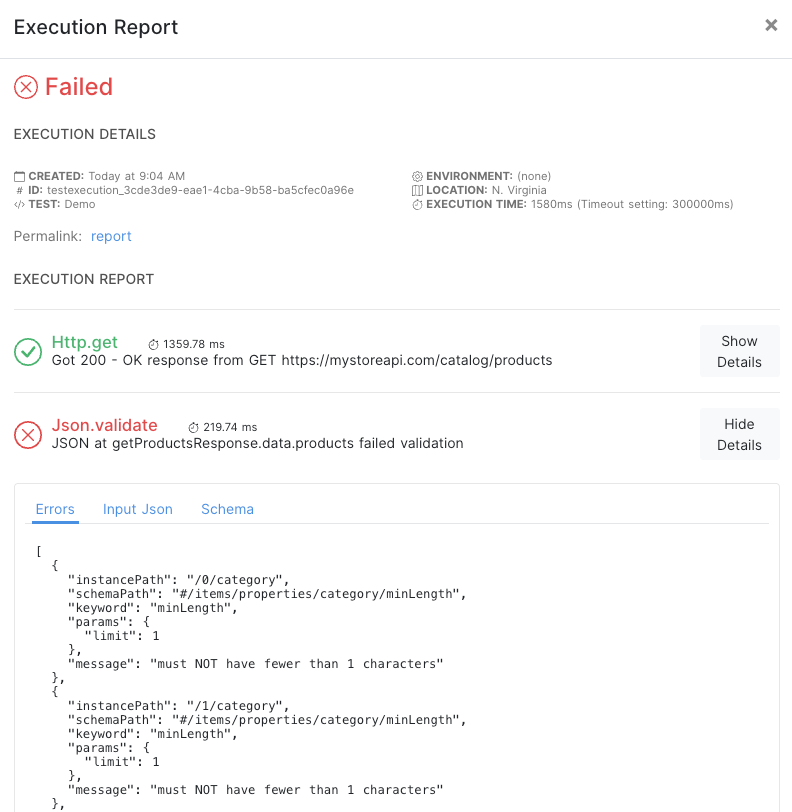
You can adjust the minLength
value to 0 or delete the field to fix this.
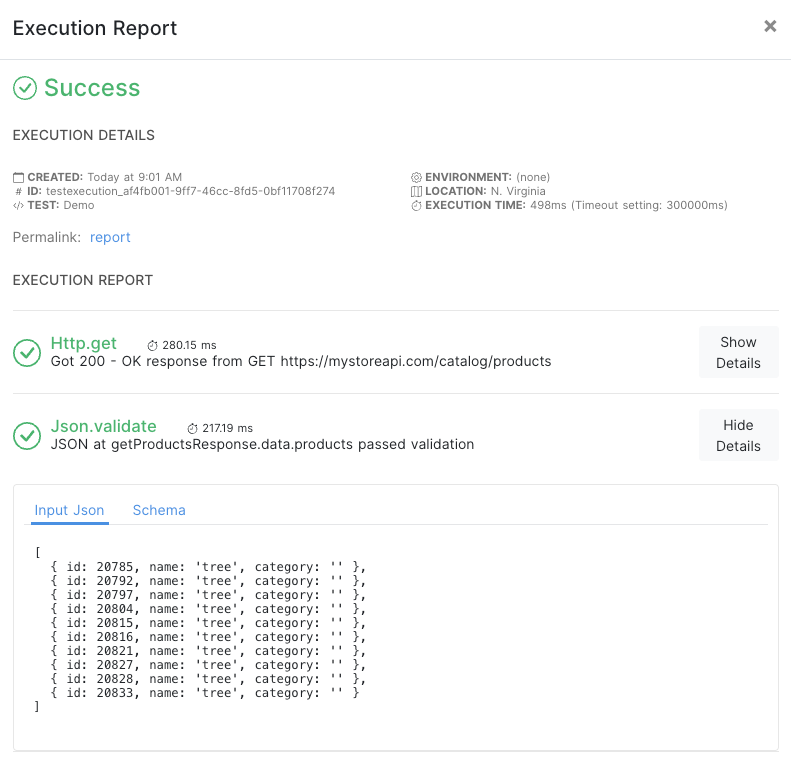
String format validation
In some instances, you may also want to validate the format of a string value in a JSON response. For example, in the response payload below, we may want to check that the date
field is always in the date-time
format.
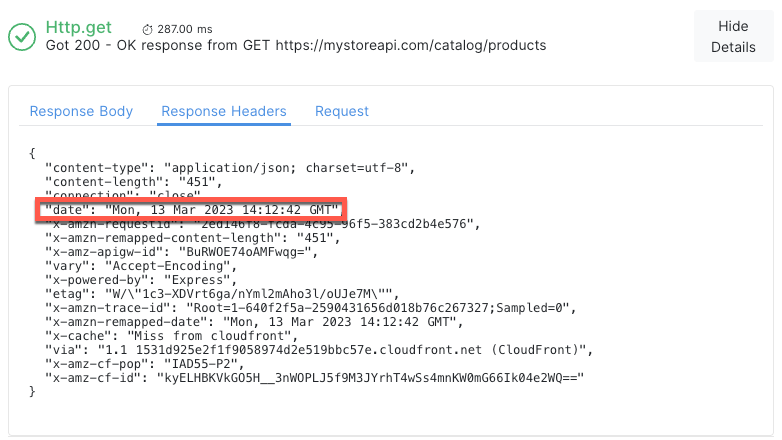
We can accomplish this via JSON Schema validation as well. You will simply need to add format
in addition to type
in your JSON Schema definition for the field. Here, we define format
as date-time
.
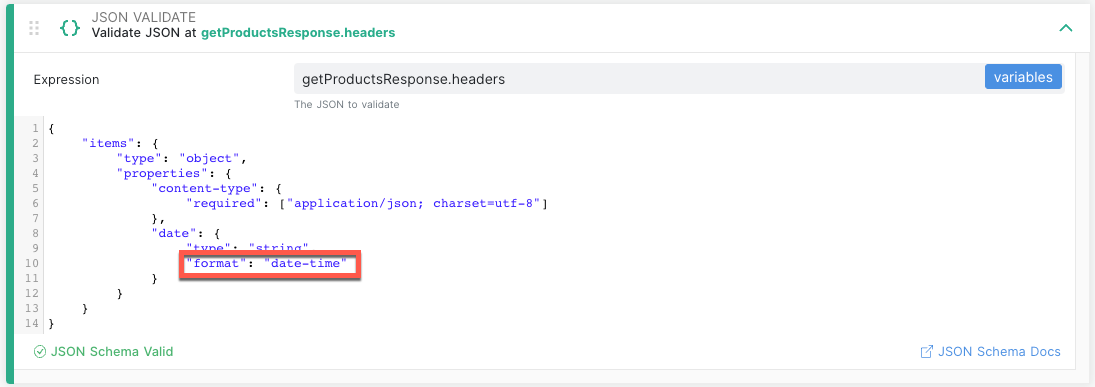
If the value is not in the date-time
format, the JSON validation will fail, and it will give you the explanation like below.
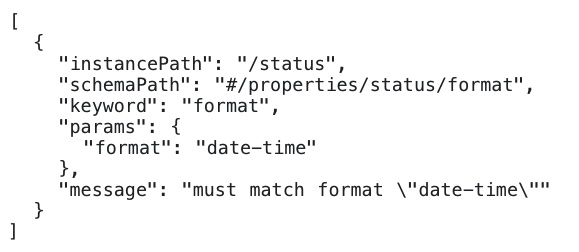
The supported string formats are listed below.
- date: full-date.
- time: time with an optional time zone.
- date-time: date-time from the same source (time-zone is mandatory).
- uri: full URI.
- email: email address.
- hostname: host name.
- ipv4: IP address v4.
- ipv6: IP address v6.
Updated over 1 year ago