Pagination (GQL)
Work with queries that return multiple items.
If you issue a query that can return multiple items, such as listing the APIs that you own, pagination information is usually returned. Pagination is used to return subsets of data, rather than all of the results in a single request. Querying for all of the data in a single request would be too expensive, does not scale well, and often is unnecessary. RapidAPI implements cursor-based pagination, as recommended on graphql.org.
A (very) little graph theory
GraphQL models your data as a graph. Graphs are common in everyday life. For example, your family tree can be modeled as a graph. A graph contains entities known as nodes (or vertices). In a family tree, the nodes are people. A graph also contains connections between the nodes, known as edges (or links). In a family tree, edges represent the relationship between the people, such as marriages or parent/child relationships.
To return results that can contain multiple items, you will often see edges and nodes in your results. Nodes contain the information that you are searching for, and edges help you obtain other information you can use to obtain more query results, because they also contain cursors. Cursors are unique strings that reference a returned item.
Pagination in the Platform API
Queries that can return multiple items often have a pagination argument. For example, the apis query includes an optional argument named pagination of type PaginationInput.
apis(
where: ApiWhereInput
orderBy: ApiOrderByInput
pagination: PaginationInput
): ApiConnection!
The PaginationInput type includes four fields, though only the first and after fields are currently used in RapidAPI queries. The value of the first field is an integer representing the maximum number of items to return. The value of the after field is a string containing a cursor of the item just prior to the the first item returned. Cursor values are obtained from previous queries. If the cursor value is not specified, the results are returned starting from the first item.
type PaginationInput {
first: Int
after: String
last: Int
before: String
}
The apis query returns an object of type ApiConnection. This type contains four fields:
- nodes - Contains an array of Api objects. It is provided as a convenience in case your current use case does not need to use paging and cursors.
- edges - Contains an array of ApiEdge objects. Each of these objects contains a node field containing an Api object, and a cursor field containing a string pointer to the current item. The value of the cursor field can be used in the after PaginationInput argument of subsequent requests. This allows you to programmatically obtain multiple pages of API information.
- pageInfo - Contains an object of type PageInfo. The PageInfo type contains four fields, though only two fields are currently used by RapidAPI. hasNextPage is a Boolean field, and contains a value of true if there are more items that can be returned. endCursor* is the String value of the pointer to the last item returned in the current query. This value can be used in the after** PaginationInput argument of subsequent requests. This allows you to programmatically obtain multiple pages of API information.
- totalCount - Contains an integer representing the total number of items that are available to be returned from the query, independent of paging information. For example, if a team owns 56 APIs, totalCount will be 56, even if only 5 APIs are returned at a time.
type ApiConnection {
nodes: [Api!]!
edges: [ApiEdge!]!
pageInfo: PageInfo!
totalCount: Int!
}
type ApiEdge {
node: Api
cursor: String
}
type PageInfo {
hasNextPage: Boolean
hasPreviousPage: Boolean
startCursor: String
endCursor: String
}
In this example, we query for the first 5 APIs owned by a user or team with an ID of 5713300.
query {
apis(
where: {
ownerId: 5713300
},
pagination: {
first: 5
},
) {
edges {
node {
id
name
}
}
pageInfo {
endCursor
hasNextPage
}
totalCount
}
}
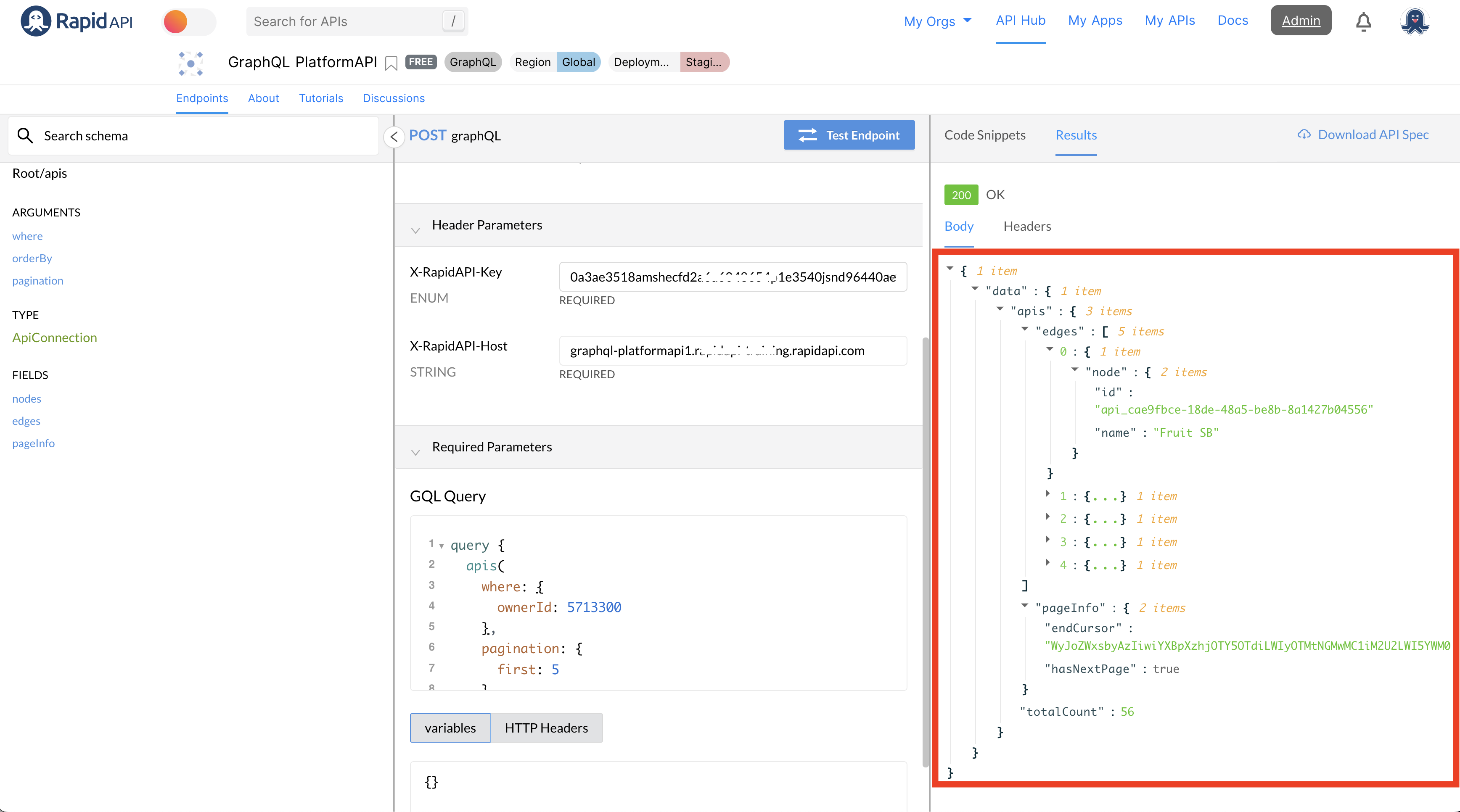
The results indicate that this owner owns 56 APIs, even though we only returned 5 APIs in the first request. We can use the value of the pageInfo.endCursor field to help obtain the second set of 5 APIs.
query {
apis(
where: {
ownerId: 5713300
},
pagination: {
first: 5
after: "WyJoZWxsbyAzIiwiYXBpXzhjOTY5OTdiLWIyOTMtNGMwMC1iM2U2LWI5YWM0M2JhMWFmZiJd"
},
) {
edges {
node {
id
name
}
}
pageInfo {
endCursor
hasNextPage
}
totalCount
}
}
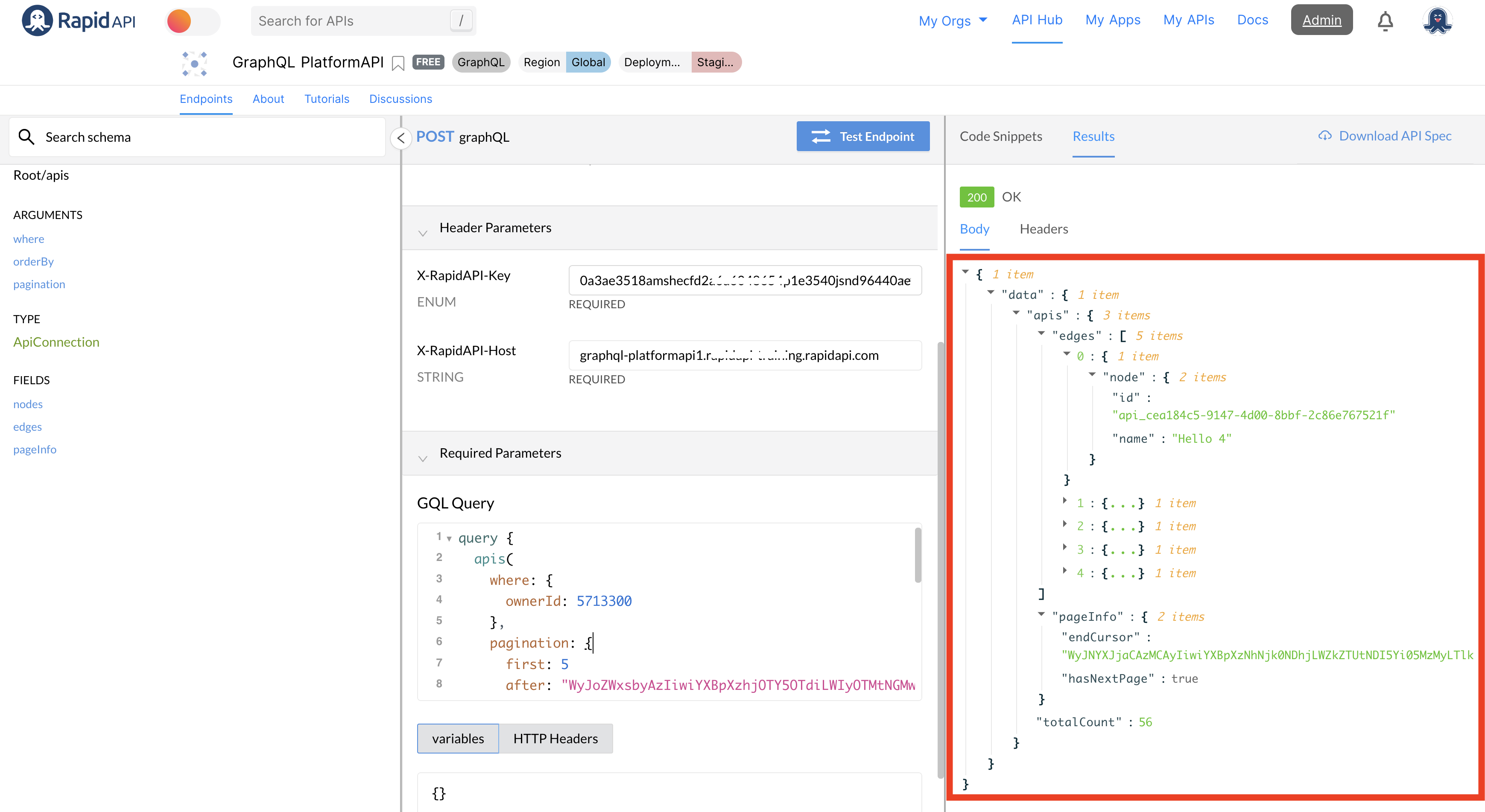
This process can continue until all of the APIs have been returned, at which point the value of pageInfo.hasNextPage will be false.
Updated about 1 month ago