JSON Schema Validation
Why validate your JSON response?
In modern software development architecture, APIs are often used as an interface for two systems to talk to each other. They can be used for frontend systems to communicate with backend logic, or simply for two backend systems to talk to each other.
Given that an API is a means of communication, a contract exists between the API builder and consumer on how the API will provide information. For the consumers to be able to seamlessly consume information, it is important that the API response always follows the API contract. Therefore, it is important to constantly monitor whether API responses meet the contract, and whether a code change that would be deployed to production would break the contract prior to deploying the change.
How can I do this in RapidAPI Testing?
Using RapidAPI Testing, you can easily validate your API responses against the schema that it needs to abide by. In order to do this, you will need the response from an API call (you can simply make the HTTP request in the test itself) and the JSON Schema (more on JSON Schema) we will validate against.
First, let’s add the HTTP request whose response you will validate. In the example below, it is simply a GET request to https://mystoreapi.com/catalog/products
. We will store the response in a variable called getProductsResponse
.
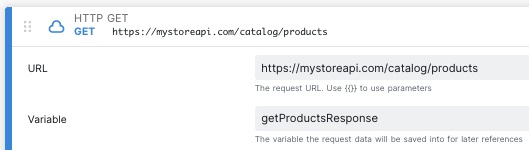
As a second step, we will add the Json validate action. Select it from the Add Step modal.
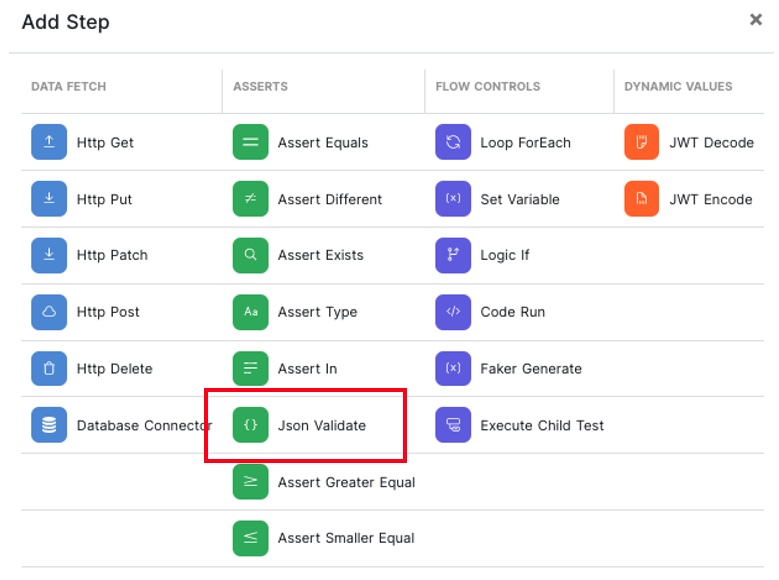
In the action that gets added to your test, enter the two pieces of information mentioned earlier. The variable that holds the JSON data we want to validate goes in the Expression field (see box 1). In this example, we want to validate the JSON in getProductsResponse.data.products
. The JSON schema we will validate against goes in the JSON schema editor (see box 2). The schema editor help you by letting you know whether the schema itself is valid or not. (More on JSON Schema).
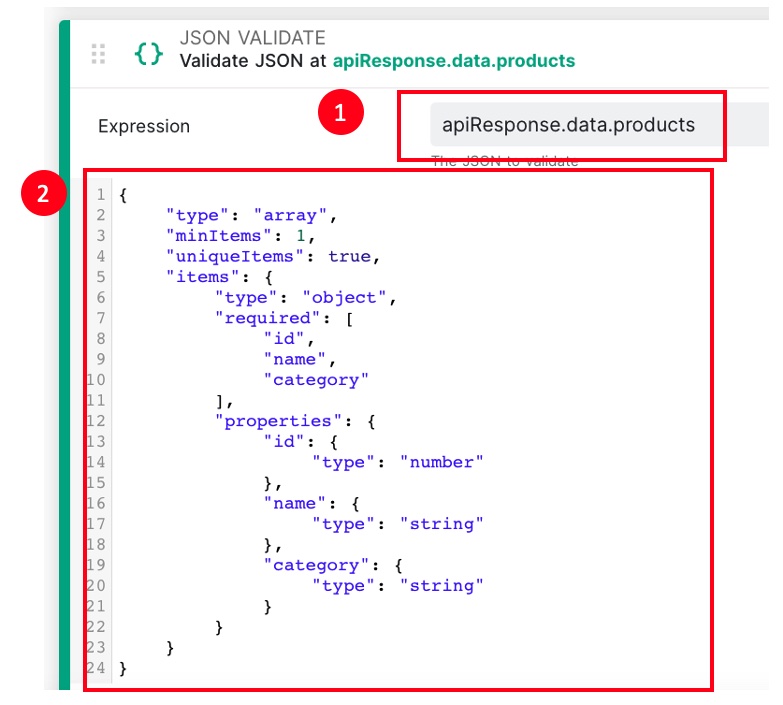
That’s it! Now, you can go ahead and execute the test. The execution report will let you know whether the JSON data provided passes the validation. If it does not, the details will explain why. In the example below, the category field needs to have at least one character but it’s an empty string.
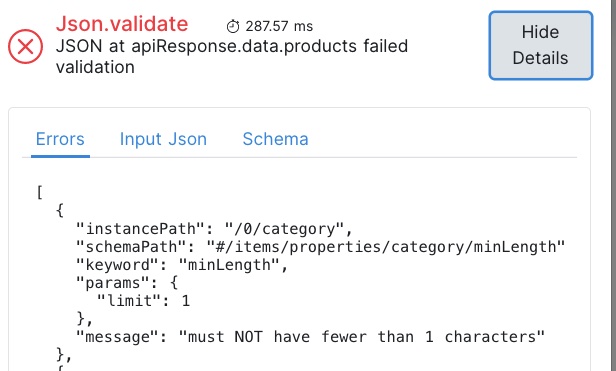
String format validation
In some instances, you may also want to validate the format of a string value in a JSON response. For example, in the response payload below, we may want to check that the edited field is always in the date-time
format.
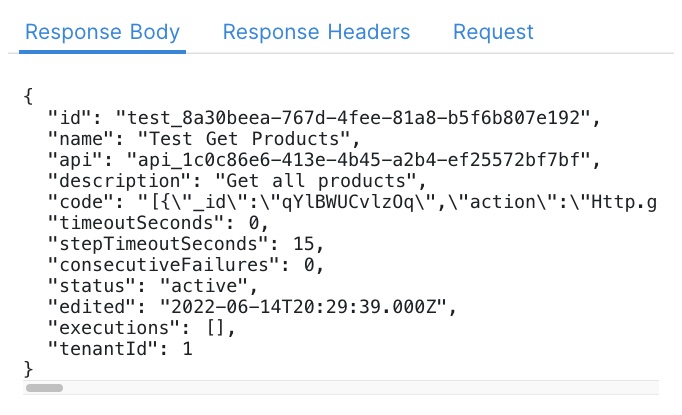
We can accomplish this via JSON Schema validation as well. You will simply need to add format
in addition to type
in your JSON Schema definition for the field. Here, we define format
as date-time
.
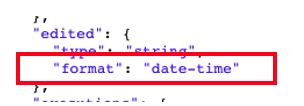
If the value is not in the date-time
format, the JSON validation will fail, and it will give you the explanation like below.
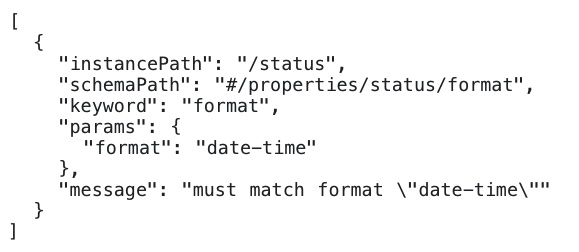
The supported string formats are listed below.
- date: full-date.
- time: time with optional time-zone.
- date-time: date-time from the same source (time-zone is mandatory).
- uri: full URI.
- email: email address.
- hostname: host name.
- ipv4: IP address v4.
- ipv6: IP address v6.
Updated over 2 years ago